Exploring New Features of Java 12
- schick09
- Jan 7
- 2 min read
Updated: Jan 20
Introduction
Java 12 brought a host of enhancements aimed at improving developer productivity, code readability, and overall application performance. From new language features like switch expressions to performance improvements in garbage collection, Java 12 continues to refine the developer experience. In this post, we’ll dive into some of the most exciting features introduced in Java 12 and explore how they can make your coding life easier.
1. How to Use Switch Expressions in Java 12
Switch expressions are a preview feature in Java 12 that simplifies conditional logic by allowing the switch statement to return a value. This feature makes code more concise and easier to read.
Before Java 12
int numLetters;
String day = "MONDAY";
switch (day) {
case "MONDAY":
case "FRIDAY":
case "SUNDAY":
numLetters = 6;
break;
case "TUESDAY":
numLetters = 7;
break;
default:
numLetters = 0;
}
With Java 12
String day = "MONDAY";
int numLetters = switch (day) {
case "MONDAY", "FRIDAY", "SUNDAY" -> 6;
case "TUESDAY" -> 7;
default -> 0;
};
This new syntax reduces boilerplate code and provides a more expressive way to handle conditional logic.
2. Shenandoah Garbage Collector (Experimental)
Shenandoah is an experimental low-pause-time garbage collector introduced in Java 12. It minimizes pause times by performing concurrent compaction of the heap.
Benefits of Shenandoah Garbage Collector in Java
Reduced pause times make applications more responsive.
Suitable for applications requiring low-latency performance, such as real-time systems.
Enabling Shenandoah
To use Shenandoah, pass the following JVM argument:
-XX:+UseShenandoahGC
3. Compact Number Formatting
The new compactNumberFormat API provides a way to format numbers in a human-readable, compact form.
Java 12 Compact Number Formatting Example
import java.text.NumberFormat;
import java.util.Locale;
public class CompactNumberExample {
public static void main(String[] args) {
NumberFormat fmt = NumberFormat.getCompactNumberInstance(Locale.US, NumberFormat.Style.SHORT);
System.out.println(fmt.format(1000)); // Output: 1K
System.out.println(fmt.format(1000000)); // Output: 1M
}
}
This API is particularly useful for financial or statistical applications where compact, readable data representation is needed.
4. Understanding G1 Garbage Collector Enhancements In Java 12
Java 12 introduced enhancements to the G1 garbage collector, which now automatically returns unused heap memory to the operating system. This optimization helps reduce the memory footprint of Java applications, making them more resource-efficient.
5. JVM Constants API
The new jdk.constant package models nominal descriptions of key class-file and runtime artifacts, enabling advanced tools to work with constant pools more effectively.
Example
The ConstantDesc API allows developers to describe constants in a structured manner, improving interoperability and readability.
6. Java 12 Support for Unicode 11 Explained
Java 12 added support for Unicode 11, which includes 684 new characters and several new scripts. This ensures better internationalization and compatibility for applications targeting global audiences.
Conclusion
Java 12 offers a mix of developer-friendly features and performance enhancements that streamline coding and improve application efficiency. Whether you’re excited about the concise switch expressions or the advanced garbage collectors, these updates demonstrate Java’s ongoing commitment to evolving with modern development needs.
If you haven’t tried Java 12 yet, now is a great time to explore its new features and see how they can enhance your projects. Which feature are you most excited about? Let us know in the comments!
Here's a link to our GitHub location to access more Java 12 code examples - https://github.com/daveschickconsulting/java_12_examples
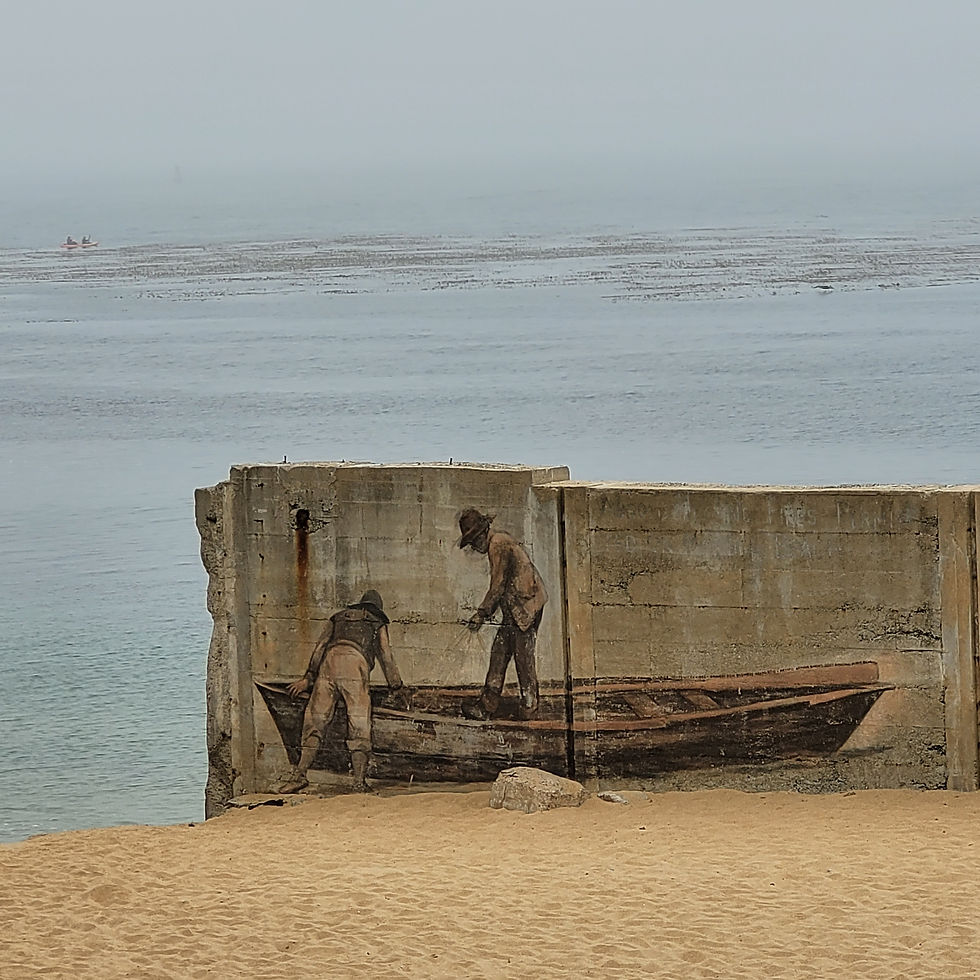
Comments